CS 111 Spring 2010 Scribe Notes
Lecture 18 (6/1/2010)
by Tim Fabian and Samuel Karp
Table of Contents
Methods for accessing system resources
- Direct
- Application has a pointer to the OS object
- Access-check is done when pointer is given to application (via virtual memory)
- + Fast access after initial check
- - Resource can be more easily corrupted
- Indirect
- Application gets an opaque handle (e.g. file descriptor)
- + Fine-grained access control (e.g. revoke access)
- - Slower access: syscall overhead
Top
Access control
Goals
- Privacy - prohibit reads by other processes
- Integrity - prohibit writes by other processes
- Trust - only run trusted code
- Sharing - get your work done
- Non-goal: DoS defense
Design concepts
- Threat analysis
- Security model
Keeping track of what access is allowed - The access control data itself should be protected, but updatable (sensitive operation).
- Controlled operation needed
- Indirect access, due to sensitivity
There are two main forms of implementing access control
- Access Control Lists (ACLs)
- Capabilities - No centralized ACL, but "keys" to access object that can be used by anyone
In both cases, access control data
- Must be unforgable (at least in part must live "in" OS)
- Must be consulted before access
- Needs HW and/or OS support
Representing ACL data
Array
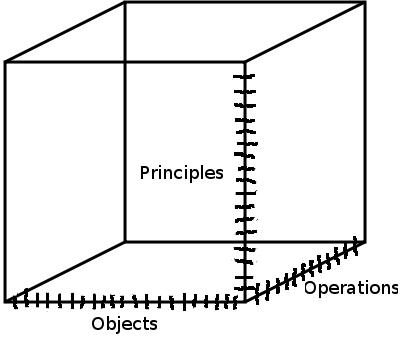
Three-dimensional array of permission bits. One axis represents Principals (processes and/or users), the second axis represents all objects, and the third axis represents operations. If the axis of principals is 1e4 bits, the axis of objects is 1e6 bits, and the axis of operations is 1e2 bits, the total storage space required for this information is 1e12 bits.
Unix permissions model
Each object has 9 permissions bits plus a user ID and group ID. If the user ID and group ID are each 32-bits, the total is 73-bits.
$ ls -l
rwxrwxrwx
- + Much more compact
- + Easy to check
- + A single process can have several groups simultaneously
- - Only room to represent three operations
- - Not all combinations are supported
- A bit too generous in many cases
- Sysadmin (root) is in charge of group membership (which can be very inflexible)
- Hard to maintain (lots of people, lots of roles
ACLs (Windows NT-style) - Now in Linux, Solaris as well
Associated with each file is a list of users (+ groups) that can access the file +flags (operations)
- syscalls such as getfacl() and setfacl() allow flexible management of the ACLs
- A bit too generous in many cases
- Hard to maintain (lots of people, lots of roles
Role Based Access Control (RBAC) - Solaris, Active Directory
Users can assume roles
- Rights are associated with roles, not users
- If you assume a role you may lose rights that you had in a previous role
- You can have >1 sessions with different roles
This often comes with fine-grained control over operations
Capabilities
Possession of this "word" implies right to object
- The "word" is not process specific - it can be shared by a process
- Capabilities can be implemented by:
- Encryption - capabilities can be sent across the net
- Index into OS table (e.g. file descriptor).
- The "word" must be wide enough so that it can not easily be guessed
- Issue of containment: the capability can escape (eg capability saved in a log file and stolen)
Top
Denial of Service (DoS) attacks
Bog a server down without enough requests to render it unable to process legitimate user requests
Defense methods
- captcha
- captchas can be routed to a website with real users
- this allows attacking bots to bypass captchas without the need for image recognition
- log IP addresses
- Hope to recognize a signature of the attack and block it accordingly
- change server IP address
- Many attackers will use the servers IP address rather than domain name
- This approach speeds up each attack, but, if the IP address changes, the attacks will fail
- make the server faster!
- The server becomes harder to bog down and performance increases for legitimate users
- There are multiple approaches for speeding up an Apache server's request handling
- Consider this simple Apache pseudocode:
for(;;) {
   fd = accept(); // listen for a connection
   req = read(fd,...); // read the request
   handle(req); // do the necessary operations to handle the request
   close(fd); // close the connection and start over
}
This simplest approach illustrates the basic request method but does so without parallelism, making the server only able to handle one request at a time
- Immediately, we may think to fork off each connection
for(;;) {
   fd = accept(); // listen for a connection
   fork(); // fork a child to handle request, parent can keep listening
   req = read(fd,...); // read the request
   handle(req); // do the necessary operations to handle the request
   close(fd); // close the connection and start over
}
This still rather simple approach is slow!
- Apache is a big program and each fork takes a long time
- If forks are too slow, the next idea might be to try multithreading
- The generic problem with multithreading, lack of isolation, can be crippling to the web server
- The server most likely has several functions for handling each request type
- If one handler function is buggy, it can crash all of the handler functions and the server!
- A commonly adopted solution is to use pre-forked children
- The idea is the same as approach #2; however, it is much faster
- Requests are passed off to processes which already exist, eliminating the fork overhead
- Additionally, this method retains the advantages of process isolation so that, if one handler fails, the system will not crash and a replacement process can be forked off
- The fastest approach, however, is an event-based server
- Such servers use multithreading (1 thread per CPU) and non-blocking I/O to efficiently handle requests
- This approach allows the server to respond to events and do its own process scheduling
- Although it's complicated, a skilled programmer can optimize the server
Top
CS 111 Operating Systems Principles, UCLA. Paul Eggert. June 1, 2010.